Introduction
Le plug-in Pulse de Brightcove vous permet d'intégrer le SDK Pulse d'Invidi au SDK natif Brightcove pour Android. Pulse est une plateforme de publicité vidéo. Pour les campagnes et les détails de configuration, consultez leur Mode d'emploi.
Étapes
Une fois qu'une campagne est créée sur la plate-forme Pulse, vous pouvez commencer à utiliser le plug-in Pulse pour le Brightcove Native SDK pour Android. Suivez ces étapes pour intégrer le plug-in Pulse à votre projet :
-
Dans votre module build.gradle , ajoutez la dépendance du plugin Pulse.
dependencies { implementation 'com.brightcove.player:android-pulse-plugin:6.12.0' }
-
Téléchargez le Fichier .aar du SDK Pulse.
-
Dans ton application/librairies dossier, ouvrez le build.gradle fichier de votre module. Modifiez les éléments suivants :
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar', '*.aar']) }
-
Dans ton MainActivity.java , initialisez le Pulse Plugin en instanciant un
PulseComponent
avec l'URL hôte Pulse créée pour votre campagne.@Override protected void onCreate(Bundle savedInstanceState) { // ... // Creating pulse component PulseComponent pulseComponent = new PulseComponent( "your pulse host url", brightcoveVideoView.getEventEmitter(), brightcoveVideoView); // ... }
-
Met le
PulseComponent
Auditeur.pulseComponent.setListener(new PulseComponent.Listener() { @NonNull @Override public PulseSession onCreatePulseSession( @NonNull String hostUrl, @NonNull Video video, @NonNull ContentMetadata contentMetadata, @NonNull RequestSettings requestSettings) { // See step 3a return Pulse.createSession(contentMetadata, requestSettings); } @Override public void onOpenClickthrough(@NonNull PulseVideoAd pulseVideoAd) { } });
-
Mettre en œuvre le
onCreatePulseSession
méthode, qui crée unePulseSession
et le renvoie auPulseComponent
. Il y a trois paramètres :- L'hôte d'impulsion
- Les paramètres de métadonnées de contenu
- Les paramètres de la demande
@NonNull @Override public PulseSession onCreatePulseSession( @NonNull String hostUrl, @NonNull Video video, @NonNull ContentMetadata contentMetadata, @NonNull RequestSettings requestSettings) { // Set the pulse Host: Pulse.setPulseHost(pulseHostUrl, null, null); // Content metadata settings contentMetadata.setCategory("skip-always"); contentMetadata.setTags(Collections.singletonList("standard-linears")); contentMetadata.setIdentifier("demo"); // Request Settings: // Adding mid-rolls List<Float> midrollCuePoints = new ArrayList<>(); midrollCuePoints.add(60f); requestSettings.setLinearPlaybackPositions(midrollCuePoints); // Create and return the PulseSession return Pulse.createSession(contentMetadata, requestSettings); }
-
Mettre en œuvre le
onOpenClickthrough
méthode, qui est appelée lorsque le apprendre encore plus bouton d'une annonce linéaire est cliqué. Une action typique pour ce rappel consiste à ouvrir le navigateur avec l'URL attendue.@Override public void onOpenClickthrough(@NonNull PulseVideoAd pulseVideoAd) { Intent intent = new Intent(Intent.ACTION_VIEW) .setData(Uri.parse(pulseVideoAd.getClickthroughURL().toString())); brightcoveVideoView.getContext().startActivity(intent); pulseVideoAd.adClickThroughTriggered(); }
-
Jouez votre contenu
Catalog catalog = new Catalog.Builder( eventEmitter, getString(R.string.account)) .setPolicy(getString(R.string.policy)) .build(); catalog.findVideoByID(getString(R.string.videoId), new VideoListener() { // Add the video found to the queue with add(). // Start playback of the video with start(). @Override public void onVideo(Video video) { brightcoveVideoView.add(video); brightcoveVideoView.start(); } });
Annonces de pause d'impulsion
Lorsque la campagne Pulse a configuré "Pause Ads", le plugin Pulse montrera à l'utilisateur lorsque le contenu est mis en pause.
La gestion des erreurs
Toutes les erreurs seront signalées au développeur à l'aide de l'événement EventType.AD_ERROR, comme indiqué ci-dessous :
eventEmitter.on(EventType.AD_ERROR, event -> {
Throwable error = event.getProperty(Event.ERROR, Throwable.class);
Log.e(TAG, "AD_ERROR: ", error);
});
Personnalisation de l'interface utilisateur
En interne, le plugin Pulse gonfle le PulseAdView
en utilisant le R.layout.pulse_ad_view
identifiant de mise en page Pour une mise en page différente, vous pouvez créer un fichier de mise en page avec le même nom et l'ajouter au res/disposition annuaire. Cela remplace la mise en page par défaut.
Utilisez les identifiants suivants pour remplacer les valeurs par défaut :
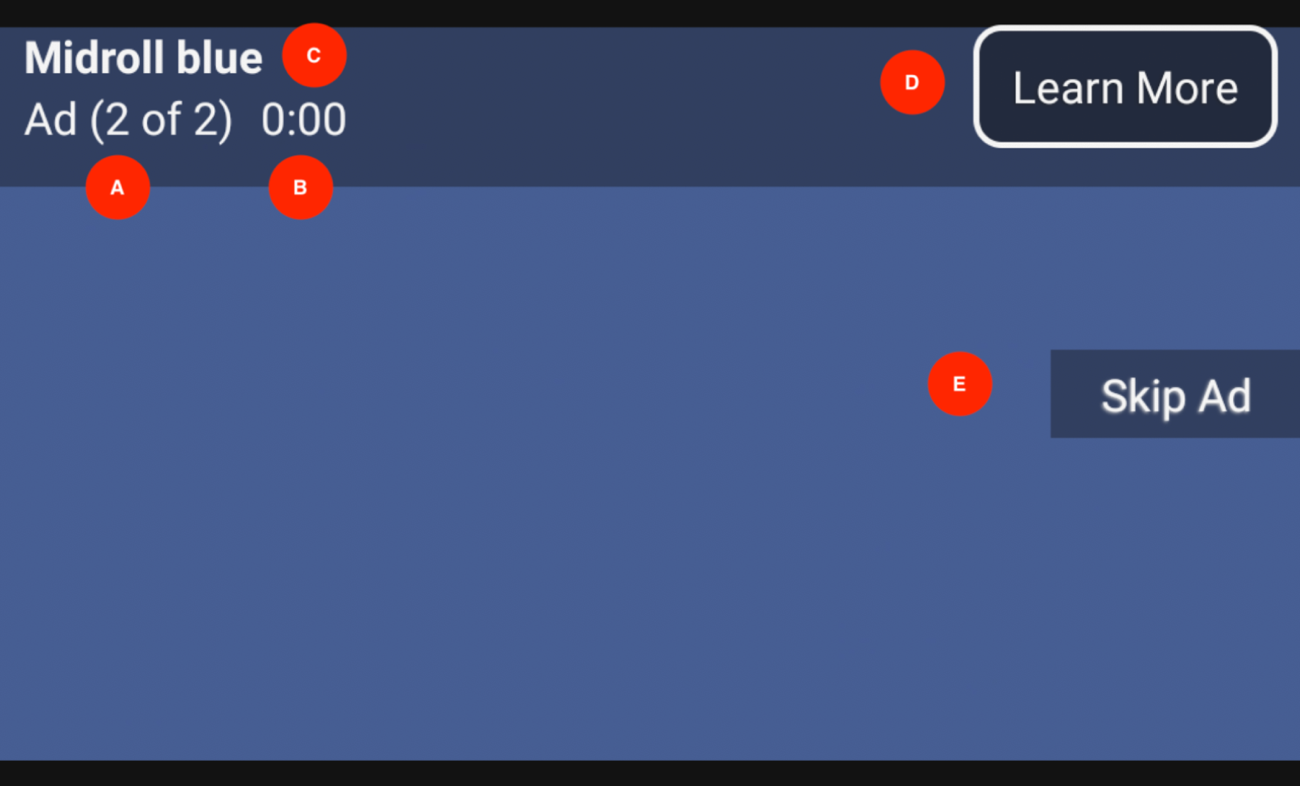
Index | Type de vue | Afficher l'identifiant |
---|---|---|
UNE | Affichage | pulse_ad_number_view |
B | Affichage | pulse_ad_countdown_view |
C | Affichage | pulse_ad_name_view |
ré | Affichage | pulse_ad_learn_more_view |
E | Affichage | pulse_skip_ad_view |
Exemple de code complet
Voici un exemple de code complet pour utiliser le plugin Pulse avec le SDK natif pour Android.
Activité
Voici un exemple du code d'activité complet :
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
final BrightcoveVideoView videoView = findViewById(R.id.video_view);
super.onCreate(savedInstanceState);
EventEmitter eventEmitter = videoView.getEventEmitter();
// Pulse setup
PulseComponent pulseComponent = new PulseComponent(
"https://pulse-demo.videoplaza.tv",
eventEmitter,
videoView);
pulseComponent.setListener(new PulseComponent.Listener() {
@NonNull
@Override
public PulseSession onCreatePulseSession(
@NonNull String pulseHostUrl,
@NonNull Video video,
@NonNull ContentMetadata contentMetadata,
@NonNull RequestSettings requestSettings) {
Pulse.setPulseHost(pulseHostUrl, null, null);
contentMetadata.setCategory("skip-always");
contentMetadata.setTags(Collections.singletonList("standard-linears"));
contentMetadata.setIdentifier("demo");
// Adding mid-rolls
List<Float> midrollCuePoints = new ArrayList<>();
midrollCuePoints.add(60f);
requestSettings.setLinearPlaybackPositions(midrollCuePoints);
return Pulse.createSession(
contentMetadata,
requestSettings);
}
@Override
public void onOpenClickthrough(@NonNull PulseVideoAd ad) {
Intent intent = new Intent(Intent.ACTION_VIEW)
.setData(Uri.parse(ad.getClickthroughURL().toString()));
videoView.getContext().startActivity(intent);
ad.adClickThroughTriggered();
}
});
Catalog catalog = new Catalog.Builder(eventEmitter, "YourAccountId")
.setPolicy("YourPolicyKey")
.build();
catalog.findVideoByID("YourVideoId", new VideoListener() {
// Add the video found to the queue with add().
// Start playback of the video with start().
@Override
public void onVideo(Video video) {
videoView.add(video);
videoView.start();
}
});
}
}
Mise en page
Voici un exemple de code de mise en page pour le R.layout.pulse_ad_view
.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:id="@+id/view_ad_details"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/pulse_skip_button_background_selector">
<TextView
android:id="@+id/pulse_ad_name_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_marginTop="4dp"
android:paddingTop="4dp"
android:paddingStart="8dp"
android:paddingEnd="8dp"
android:textColor="@color/white"
android:background="@color/bmc_live"
android:textStyle="bold"
tools:text="Preroll blue"/>
<TextView
android:id="@+id/pulse_ad_number_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingStart="8dp"
android:paddingEnd="4dp"
android:paddingBottom="4dp"
android:layout_marginBottom="8dp"
android:layout_below="@id/pulse_ad_name_view"
android:textColor="@color/white"
android:background="@color/white_semi_trans"
tools:text="Ad (1 of 2)"/>
<TextView
android:id="@+id/pulse_ad_countdown_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingStart="4dp"
android:paddingEnd="4dp"
android:paddingBottom="4dp"
android:layout_marginBottom="4dp"
android:layout_below="@id/pulse_ad_name_view"
android:layout_toEndOf="@+id/pulse_ad_number_view"
android:textColor="@color/green_almost_opaque"
android:text=""
tools:text="00:06"/>
<TextView
android:id="@+id/pulse_ad_learn_more_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="@dimen/pulse_ad_learn_more_margin_left"
android:layout_marginTop="@dimen/pulse_ad_learn_more_margin_top"
android:layout_marginEnd="@dimen/pulse_ad_learn_more_margin_right"
android:layout_marginBottom="@dimen/pulse_ad_learn_more_margin_bottom"
android:layout_alignTop="@id/pulse_ad_name_view"
android:layout_alignBottom="@id/pulse_ad_countdown_view"
android:layout_alignParentEnd="true"
android:background="@drawable/pulse_learn_more_button_background"
android:paddingStart="12dp"
android:paddingEnd="12dp"
android:padding="@dimen/pulse_ad_learn_more_padding_default"
android:gravity="center"
android:shadowColor="@color/brightcove_semitransparent"
android:shadowDx="-1"
android:shadowDy="1"
android:shadowRadius="1.5"
android:text="@string/pulse_message_learn_more"
android:textColor="@color/pulse_button_text_color"
android:nextFocusUp="@id/pulse_skip_ad_view"
android:textSize="@dimen/pulse_message_text_size"
android:visibility="gone"
tools:visibility="visible" />
</RelativeLayout>
<TextView
android:id="@+id/pulse_skip_ad_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:maxWidth="164dp"
android:layout_alignParentEnd="true"
android:layout_centerVertical="true"
android:layout_marginBottom="@dimen/pulse_skip_ad_margin_bottom"
android:background="@drawable/pulse_skip_button_background_selector"
android:ellipsize="none"
android:gravity="center"
android:maxLines="2"
android:paddingStart="@dimen/pulse_skip_ad_padding_left"
android:paddingEnd="@dimen/pulse_skip_ad_padding_right"
android:paddingTop="@dimen/pulse_skip_ad_padding"
android:paddingBottom="@dimen/pulse_skip_ad_padding"
android:scrollHorizontally="false"
android:shadowColor="@color/brightcove_shadow"
android:shadowDx="-1"
android:shadowDy="1"
android:shadowRadius="1.5"
android:text="@string/pulse_message_skip_ad"
android:textColor="@color/pulse_button_text_color"
android:textSize="@dimen/pulse_message_text_size"
android:visibility="gone"
android:nextFocusUp="@id/pulse_ad_learn_more_view"
android:focusable="true"
tools:visibility="visible"/>
</RelativeLayout>